Share this article:
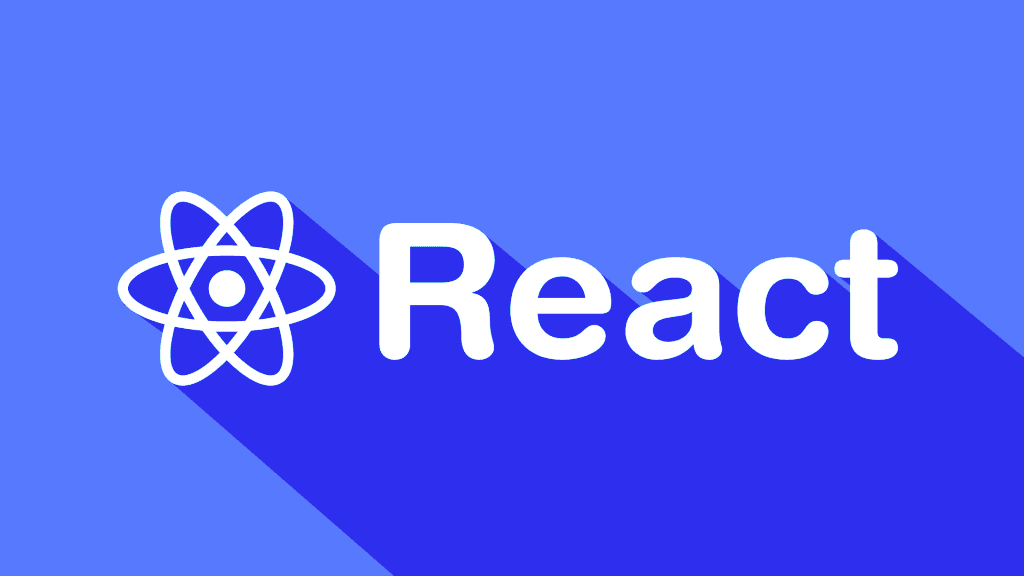
What is React
Based on the official documentation definition, React is a declarative, efficient, and flexible Javascript library for building composable user interfaces. It encourages the creation of reusable UI components that present data that changes over time.
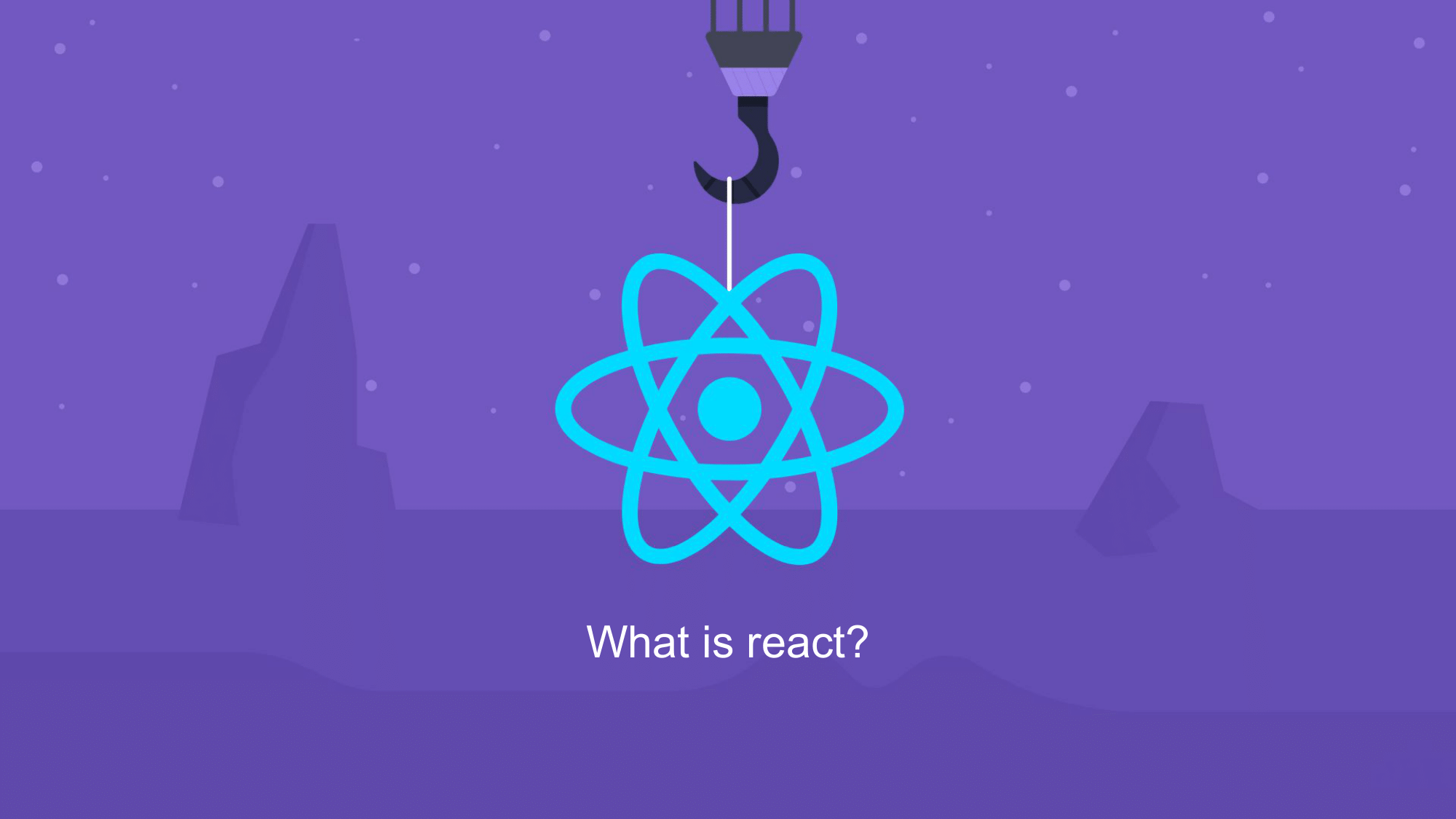
React is all about building modern, reactive user interfaces for the web. That means building single-page applications. You might have heard of the abbreviation SPA, which stands for single-page application. This means that instead of the old traditional approach where each page is loaded by the client, making requests to the server, and the server returns the loaded page, where everything is connected to the UI. Everything connected to the UI happens on the client-side with JavaScript. But why React instead of just using pure JavaScript? Let's look at an example of how we can build an app where we have to list cards and can delete a to-do list with a dialogue that prompts us to confirm the deletion. We'll compare the solutions in pure JavaScript and using React. In the example on the left using Javascript, we see the imperative solution where we build our app step by step. We need to specify every single step. For example, we create a button, attach an event listener to this button, and so on and so forth. The declarative programming approach, on the other hand, is a way of writing code that hides the actual implementation using obstructions. You use react elements to tell React which functions to invoke when rendering each component, but the library then decides when to invoke them, which makes the rendering of components declarative. Now that we have created our component once, we can reuse it elsewhere in the app with just a single line of code, and in the example presented here, we can create multiple to-do card components completely seamlessly.
Most commonly used features in REACT
React functional components
In order to construct the UI interaction, we use functional components. Components let us split the UI into independent, reusable pieces and think about each piece in isolation. A functional component is just a plain Javascript function that accepts props as an argument and returns a React element. We write components using an extension of JavaScript, which we call JSX. JSX allows us to incorporate Javascript expressions into HTML expressions. This is basically just JavaScript code that returns a value. Components in REACT can be nested into other components, and the component tree in REACT starts with an app component as the main parent, which nets other components as children.
REACT hooks
REACT hooks are functions that let us hook into REACT state and lifecycle features from function components. With hooks, you can extract stateful logic from components so it can be tested independently and reused. Hooks allow us to reuse stateful logic without changing the component hierarchy, and this makes it easy to share hooks among many components or with the community. There are some other rules to follow when using hooks as well.
- Hooks only run the scope of components and they're always coded in the top level of the REACT function. All hooks start with the 'use' word.
We're going to look into some of the hooks here that are presented:
- 'useState'
- 'useContext'
- 'useEffect'
- 'useRef'
'useState' hook
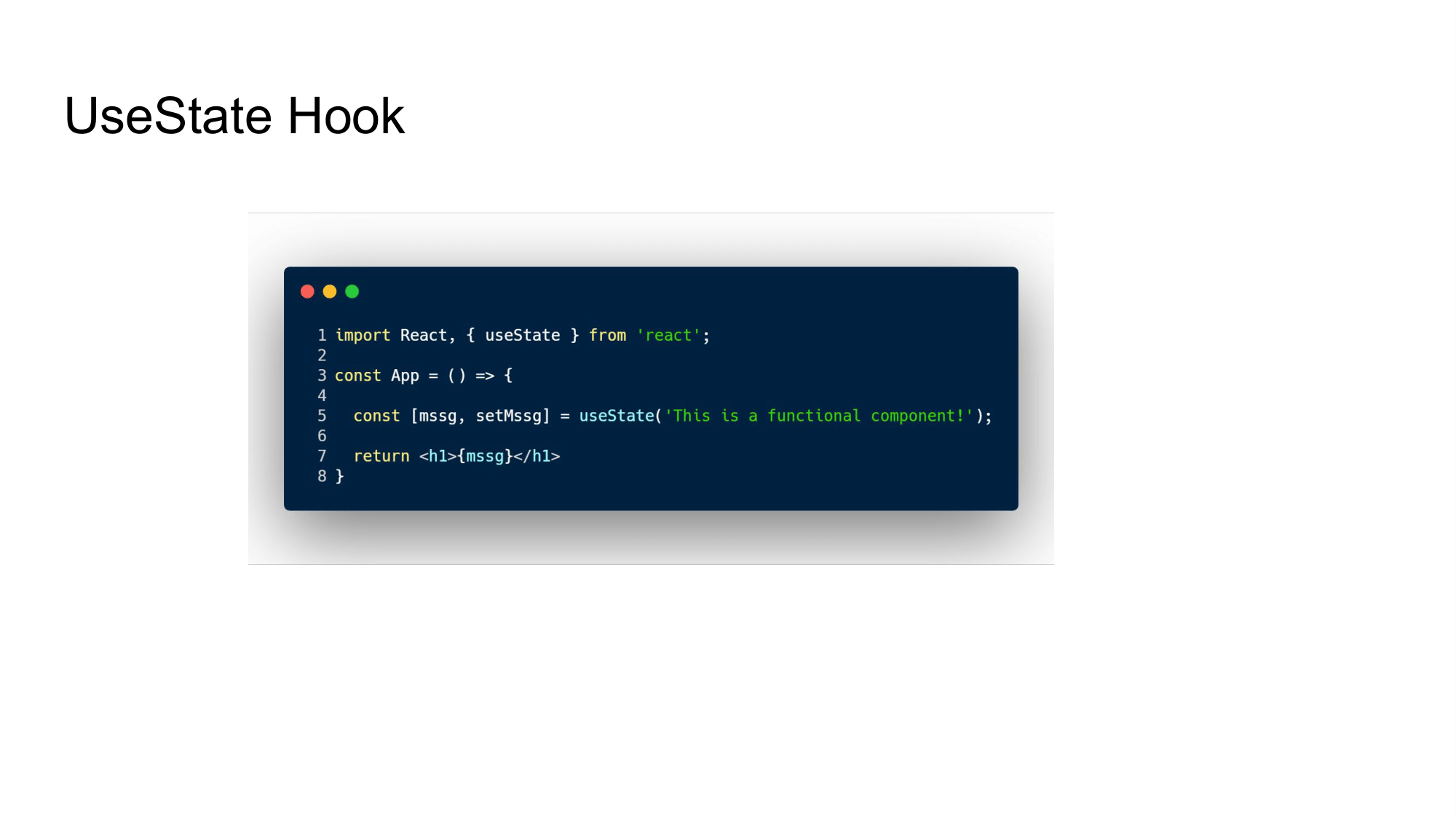
Let's start with one of the most commonly used, which is the 'useState'. 'useState' is a hook that allows us to have state variables in functional components. You pass the initial state to this function, and it returns a variable with the current state value and another function to update this value. What is important here to mention about the 'useState' is that every time we update its value, the components will render, which means that the change will be immediately shown on screen. We can also use those state variables created with the 'useState' hook and pass them down to nested children and components. This approach is called "property link" and it's one of the most common ways you come across to share data between components. There are, of course, other ways of managing state within our app. When you have a large dome tree with lots of components, it becomes cumbersome to pass data props variables to sibling components. Using context still allows us to store data in a single location, but it doesn't require us to pass the data through a bunch of components that don't need it. Of course, there is a downside to this because it means that components become less reusable. There are also other methods we can use. For example, third-party libraries. One of them is Redux. Redux allows us to manage state in a single place and keep changing our app to be more predictable and traceable. It makes the current changes in the app easier to figure out, but unfortunately, all of these benefits come with specific constraints and trade-offs. Frequently, developers feel using redux adds up some boilerplate code, making little things seem overwhelming. However, this depends solely on the app's architectural decision.
'useEffect' hook
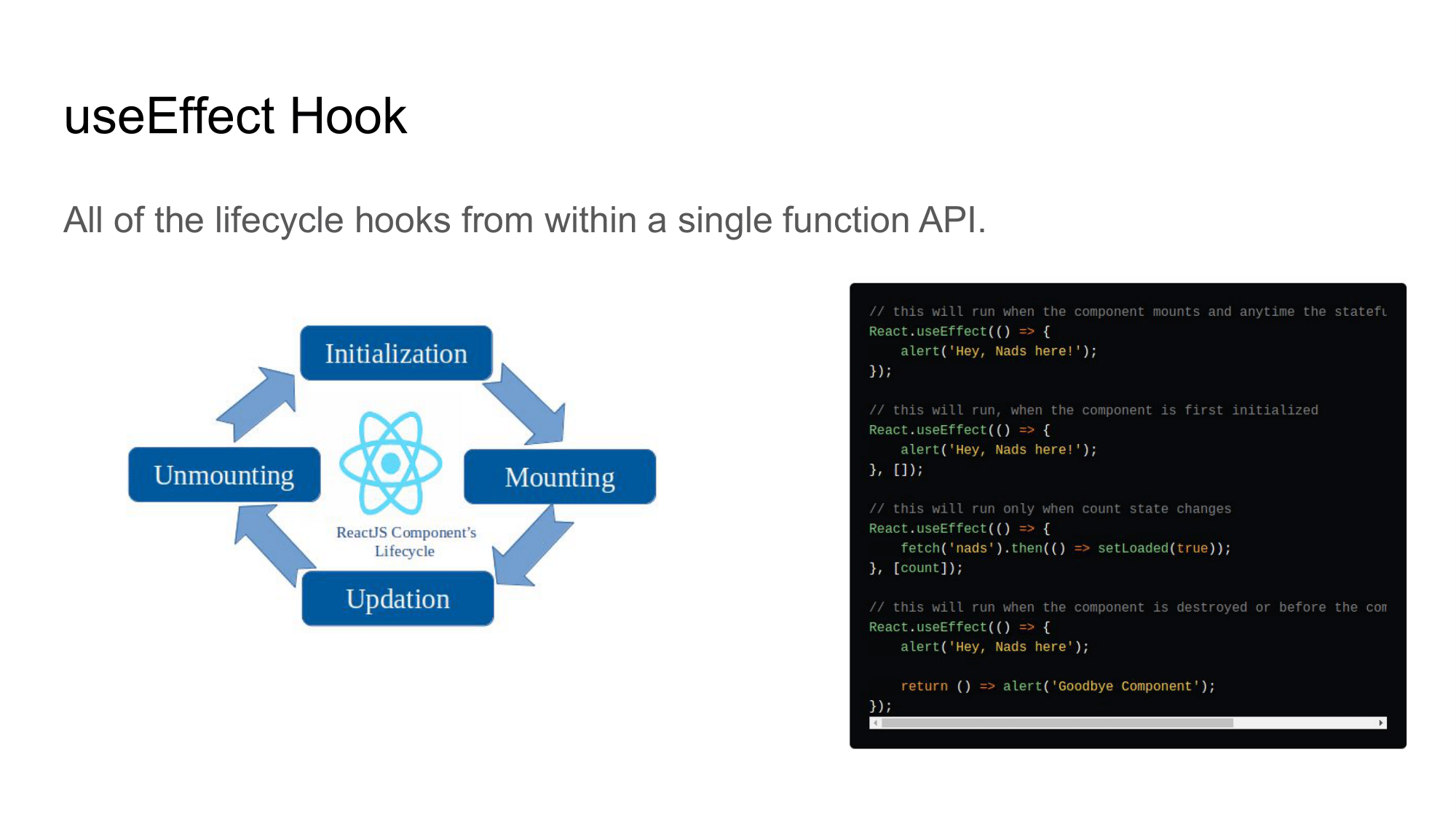
The 'useEffect' hook lets us perform side effects in function components—data fetching, setting up a subscription, and manually changing the dom in react components are all examples of such side effects. You can call this operation just side effects. In React we have something called the react components life cycle which is a collection of react component life cycle stages. With 'useEffect' hook, we can execute code for each of those life cycle stages.
So let's look into some of them:
- The initialisation in the mounting is the first stage of the React components' life cycle where the component is created and inserted into the dom tree.
- Updation can happen when a state variable is updated.
- Unmounting is when the component is removed from the dom.
'useRef' hook
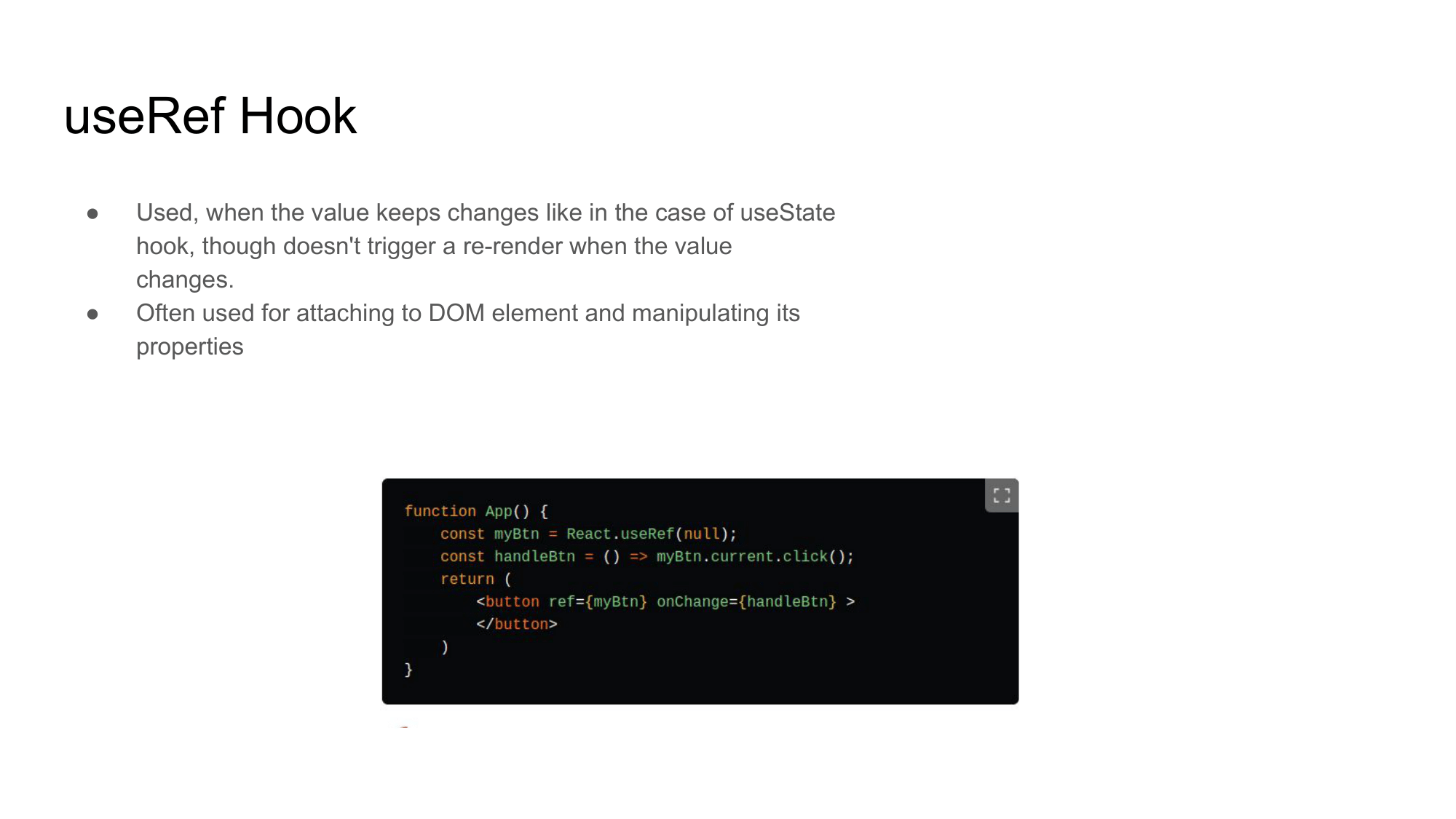
'useRef' hook is used when we want to keep the value that changes. Like in the case of the 'useState' hook, though, doesn't trigger a re-render when the value changes. Often we also use the 'useRef' hook to attach to a dom element, and then we can manipulate these properties.
Custom hooks
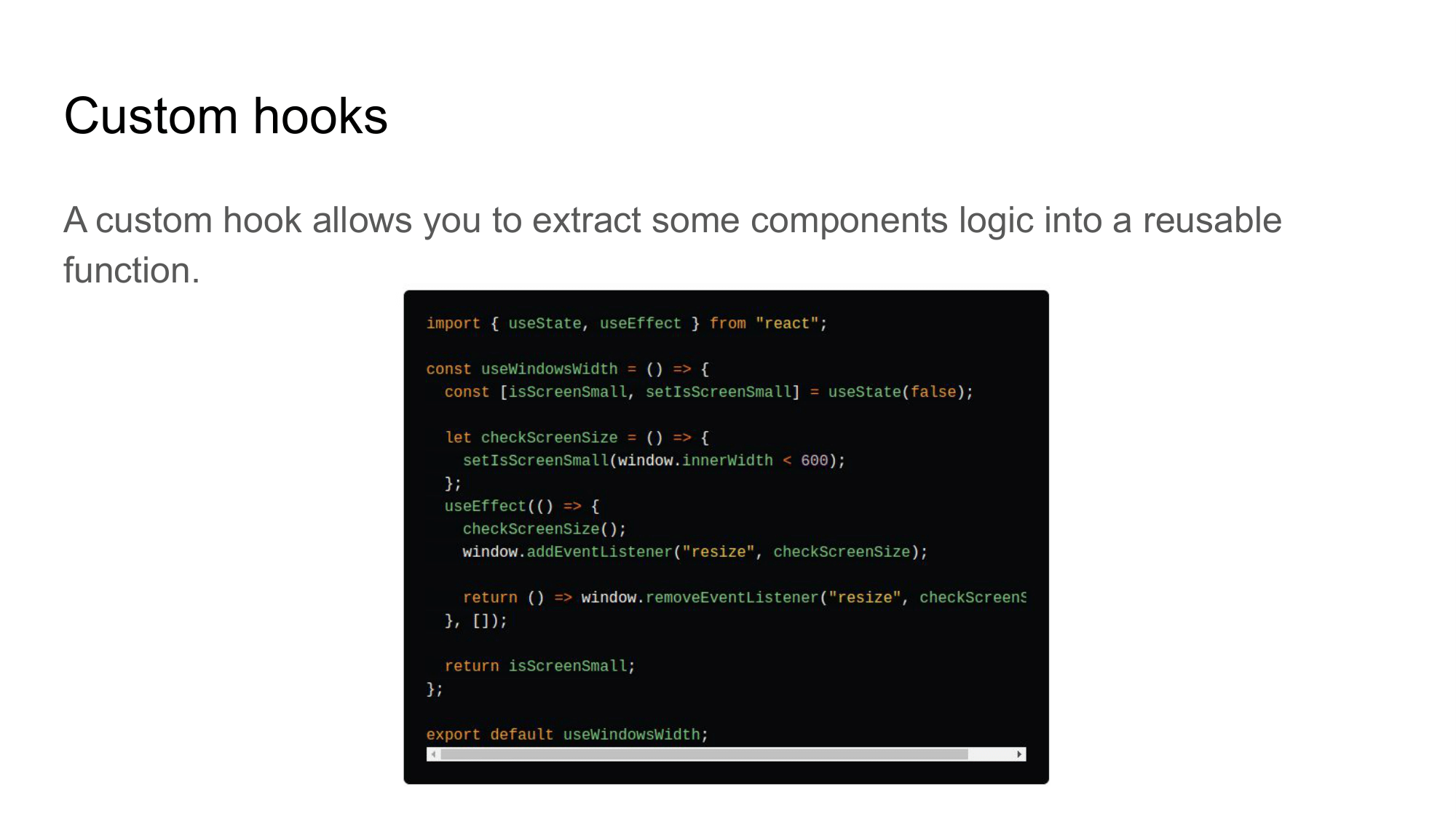
We can also create custom hooks when we want to share logic between JavaScript functional components and we can also use hooks, so we can basically use the 'useState' and 'useEffect' hooks when we create our own custom hooks.
Common reading resources
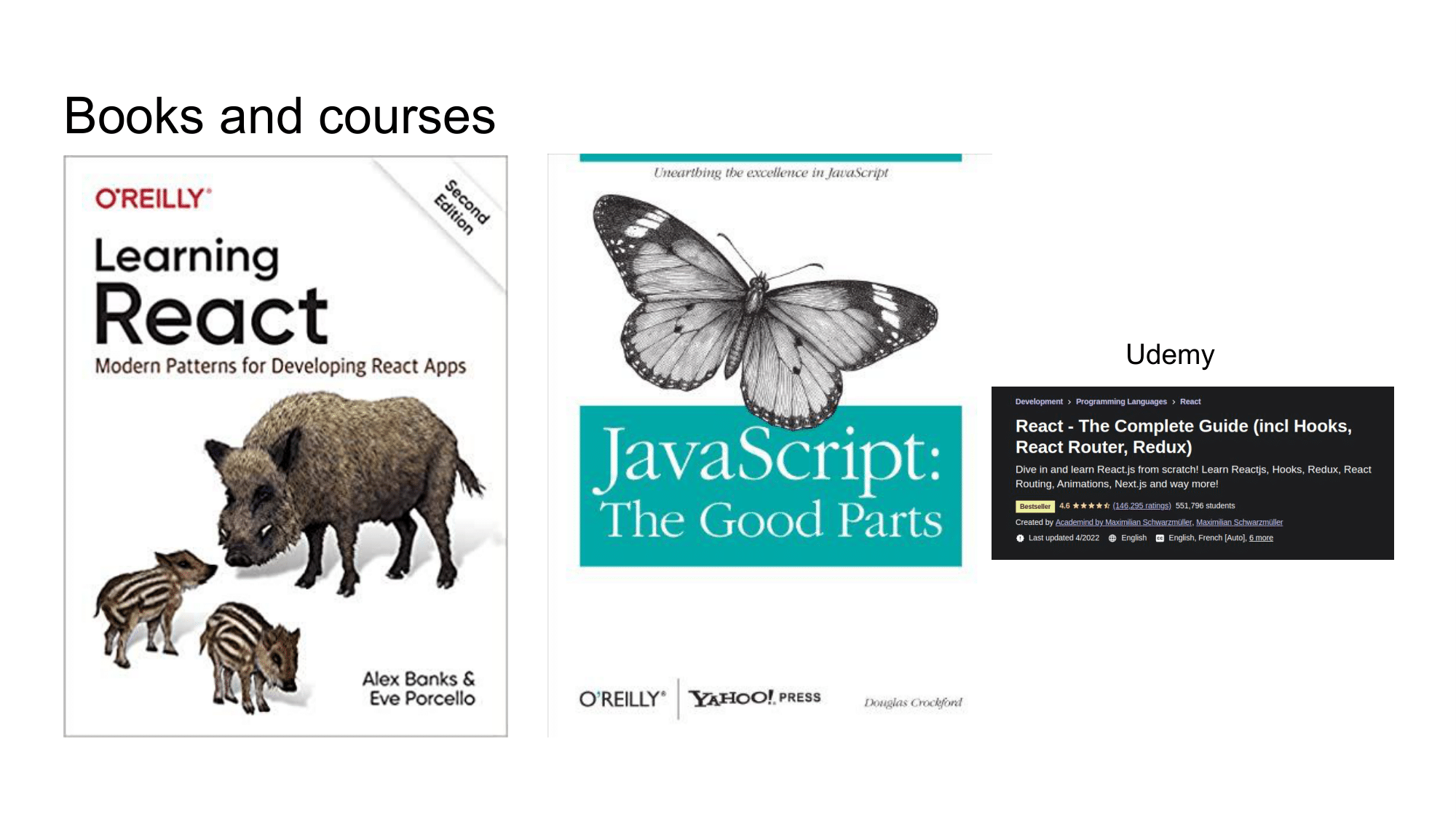
- Useful books for beginners:
- Learning React Modern Patterns For Developing React Apps
- JavaScript: The Good Parts
- Online courses that provide great structure and explanation
- Functional programming with Javascript would help you understand more about how and why we build modern React apps the way we do.
SUBSCRIBE TO OUR NEWSLETTER
Share this article: